Perl 5 pragmas
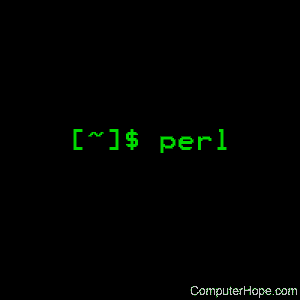
The Perl programming language offers expanded control of the interpreter by use of pragmas, directives that change the way programs and data are interpreted. This page describes pragmas in Perl 5, and how to use them.
Description
In perl (as in all programming languages), a "pragma" is a directive that specifies how the compiler (or in perl's case, the interpreter) should process its input. They are not part of the language per se, but are a sort of command-line option that tells the interpreter how to behave. The following is a list of the pragmas available in perl:
pragma | description |
---|---|
attributes | Gets/sets subroutine or variable attributes. |
autodie | Replaces functions with ones that succeed or die with lexical scope. |
autouse | Postpones the loading of modules until a function is used. |
base | Establishes an ISA relationship with base classes at compile time. |
bigint | Transparent BigInteger support for Perl. |
bignum | Transparent BigNumber support for Perl. |
bigrat | Transparent BigNumber/BigRational support for Perl. |
blib | Use MakeMaker's uninstalled version of a package. |
bytes | Forces byte semantics rather than character semantics. |
charnames | Controls access to Unicode character names and named character sequences; also used to define character names. |
constant | Declares constants. |
diagnostics | Produces verbose warning diagnostics. |
encoding | Lets you to write your script in non-ASCII or non-UTF8. |
feature | Enables new features. |
fields | Defines compile-time class fields. |
filetest | Controls the filetest permission operators. |
if | Uses a perl module if a condition holds. |
integer | Instructs the interpreter to use integer arithmetic instead of floating point. |
less | Requests "less" of a system resource. |
lib | Manipulates @INC at compile time. |
locale | Uses or avoids POSIX locales for built-in operations. |
mro | Defines method resolution order. |
open | Sets default PerlIO layers for input and output. |
ops | Restricts unsafe operations when compiling. |
overload | Used to overload perl operators. |
overloading | Lexically controls the overloading of operators. |
parent | Establishes an ISA relationship with base classes at compile time. |
re | Alters regular expression behavior. |
sigtrap | Enables simple signal handling. |
sort | Controls sort() behavior. |
strict | Restricts unsafe constructs. |
subs | Pre-declares subroutine names. |
threads | Controls interpreter-based threads. |
threads::shared | Shares data structures between threads. |
utf8 | Enables/disables UTF-8 (or UTF-EBCDIC) in source code. |
vars | Pre-declares global variable names. |
vmsish | Controls VMS-specific language features. |
warnings | Controls optional warnings. |
warnings::register | The warnings import function. |
attributes
The attributes pragma gets or sets subroutines or variable attributes.
Example
sub foo : method ; my ($x,@y,%z) : Bent = 1; my $s = sub : method { ... }; use attributes (); # optional, to get subroutine declarations my @attrlist = attributes::get(\&foo); use attributes 'get'; # import the attributes::get subroutine my @attrlist = get \&foo;
autodie
The autodie pragma replaces functions with ones that succeed or die with lexical scope.
Example
use autodie; # Recommended: implies 'use autodie qw(:default)' use autodie qw(:all); # Recommended more: defaults and system/exec. use autodie qw(open close); # open/close succeed or die open(my $fh, "<", $filename); # No need to check! { no autodie qw(open); # open failures won't die open(my $fh, "<", $filename); # Could fail silently! no autodie; # disable all autodies }
autouse
The autouse pragma postpones the loading of modules until a function is used.
Example
use autouse 'Carp' => qw(carp croak); carp "this carp was predeclared and autoused ";
base
The base pragma establishes an ISA relationship with base classes at compile time.
Example
package Baz; use base qw(Foo Bar);
bigint
The bigint pragma offers transparent BigInteger support for Perl.
Example
use bigint; $x = 2 + 4.5,"\n"; # BigInt 6 print 2 ** 512,"\n"; # really is what you think it is print inf + 42,"\n"; # inf print NaN * 7,"\n"; # NaN print hex("0x1234567890123490"),"\n"; # Perl v5.10.0 or later { no bigint; print 2 ** 256,"\n"; # a normal Perl scalar now } # Import into current package: use bigint qw/hex oct/; print hex("0x1234567890123490"),"\n"; print oct("01234567890123490"),"\n";
bignum
The bignum pragma offers transparent BigNumber support for Perl.
Example
use bignum; $x = 2 + 4.5,"\n"; # BigFloat 6.5 print 2 ** 512 * 0.1,"\n"; # really is what you think it is print inf * inf,"\n"; # prints inf print NaN * 3,"\n"; # prints NaN { no bignum; print 2 ** 256,"\n"; # a normal Perl scalar now } # for older Perls, import into current package: use bignum qw/hex oct/; print hex("0x1234567890123490"),"\n"; print oct("01234567890123490"),"\n";
bigrat
The bigrat pragma offers transparent BigNumber/BigRational support for Perl.
Example
use bigrat; print 2 + 4.5,"\n"; # BigFloat 6.5 print 1/3 + 1/4,"\n"; # produces 7/12 { no bigrat; print 1/3,"\n"; # 0.33333... } # Import into current package: use bigrat qw/hex oct/; print hex("0x1234567890123490"),"\n"; print oct("01234567890123490"),"\n";
blib
The blib pragma uses MakeMaker's uninstalled version of a package.
Example
perl -Mblib script [args...] perl -Mblib=dir script [args...]
bytes
The bytes pragma forces byte semantics rather than character semantics.
Example
use bytes; ... chr(...); # or bytes::chr ... index(...); # or bytes::index ... length(...); # or bytes::length ... ord(...); # or bytes::ord ... rindex(...); # or bytes::rindex ... substr(...); # or bytes::substr no bytes;
charnames
The charnames pragma accesses Unicode character names and named character sequences; it also defines character names.
Example
use charnames ':full'; print "\N{GREEK SMALL LETTER SIGMA} is called sigma.\n"; print "\N{LATIN CAPITAL LETTER E WITH VERTICAL LINE BELOW}", " is an officially named sequence of two Unicode characters\n"; use charnames ':loose'; print "\N{Greek small-letter sigma}", "can be used to ignore case, underscores, most blanks," "and when you aren't sure if the official name has hyphens\n"; use charnames ':short'; print "\N{greek:Sigma} is an upper-case sigma.\n"; use charnames qw(cyrillic greek); print "\N{sigma} is Greek sigma, and \N{be} is Cyrillic b.\n"; use utf8; use charnames ":full", ":alias" => { e_ACUTE => "LATIN SMALL LETTER E WITH ACUTE", mychar => 0xE8000, # Private use area "自転車に乗る人" => "BICYCLIST" }; print "\N{e_ACUTE} is a small letter e with an acute.\n"; print "\N{mychar} allows me to name private use characters.\n"; print "And I can create synonyms in other languages,", " such as \N{自転車に乗る人} for "BICYCLIST (🚴, U+1F6B4)\n"; use charnames (); print charnames::viacode(0x1234); # prints "ETHIOPIC SYLLABLE SEE" printf "%04X", charnames::vianame("GOTHIC LETTER AHSA"); # prints # "10330" print charnames::vianame("LATIN CAPITAL LETTER A"); # prints 65 on # ASCII platforms; # 193 on EBCDIC print charnames::string_vianame("LATIN CAPITAL LETTER A"); # prints "A"
constant
The constant pragma is used to declare constants.
Example
use constant PI => 4 * atan2(1, 1); use constant DEBUG => 0; print "Pi equals ", PI, "...\n" if DEBUG; use constant { SEC => 0, MIN => 1, HOUR => 2, MDAY => 3, MON => 4, YEAR => 5, WDAY => 6, YDAY => 7, ISDST => 8, }; use constant WEEKDAYS => qw( Sunday Monday Tuesday Wednesday Thursday Friday Saturday ); print "Today is ", (WEEKDAYS)[ (localtime)[WDAY] ], ".\n";
diagnostics
The diagnostics pragma and splain standalone filter program produce verbose warning diagnostics.
Example
Using the diagnostics pragma:
use diagnostics; use diagnostics -verbose; enable diagnostics; disable diagnostics;
Using the splain standalone filter program:
perl program 2>diag.out splain [-v] [-p] diag.out
Using diagnostics to get stack traces from a misbehaving script:
perl -Mdiagnostics=-traceonly my_script.pl
encoding
The encoding pragma lets you write your script in non-ascii or non-utf8.
This module is deprecated under perl 5.18. It uses a mechanism provided by perl that is deprecated under 5.18 and higher, and may be removed in a future version.
Example
use encoding "greek"; # Perl like Greek to you? use encoding "euc-jp"; # Jperl! # or you can even do this if your shell supports your native encoding perl -Mencoding=latin2 -e'...' # Feeling centrally European? perl -Mencoding=euc-kr -e'...' # Or Korean? # more control # A simple euc-cn => utf-8 converter use encoding "euc-cn", STDOUT => "utf8"; while(<>){print}; # "no encoding;" supported (but not scoped!) no encoding; # an alternate way, Filter use encoding "euc-jp", Filter=>1; # now you can use kanji identifiers -- in euc-jp! # switch on locale - # note that this probably means that unless you have complete control # over the environments the application is ever going to run in, you should # NOT use the feature of encoding pragma allowing you to write your script # in any recognized encoding because changing locale settings wrecks # the script; you can of course still use the other features of the pragma. use encoding ':locale';
feature
The feature pragma is used to enable new features.
Example
use feature qw(say switch); given ($foo) { when (1) { say "\$foo == 1" } when ([2,3]) { say "\$foo == 2 || \$foo == 3" } when (/^a[bc]d$/) { say "\$foo eq 'abd' || \$foo eq 'acd'" } when ($_ > 100) { say "\$foo > 100" } default { say "None of the above" } } use feature ':5.10'; # loads all features available in perl 5.10 use v5.10; # implicitly loads :5.10 feature bundle
fields
The fields pragma defines compile-time class fields.
Example
{ package Foo; use fields qw(foo bar _Foo_private); sub new { my Foo $self = shift; unless (ref $self) { $self = fields::new($self); $self->{_Foo_private} = "this is Foo's secret"; } $self->{foo} = 10; $self->{bar} = 20; return $self; } } my $var = Foo->new; $var->{foo} = 42; # this will generate an error $var->{zap} = 42; # subclassing { package Bar; use base 'Foo'; use fields qw(baz _Bar_private); # not shared with Foo sub new { my $class = shift; my $self = fields::new($class); $self->SUPER::new(); # init base fields $self->{baz} = 10; # init own fields $self->{_Bar_private} = "this is Bar's secret"; return $self; } }
filetest
The filetest pragma controls the filetest permission operators.
Example
$can_perhaps_read = -r "file"; # use the mode bits { use filetest 'access'; # intuit harder $can_really_read = -r "file"; } $can_perhaps_read = -r "file"; # use the mode bits again
if
The if pragma uses a Perl module if a condition holds.
Example
use if CONDITION, MODULE => ARGUMENTS;
integer
The integer pragma tells perl to use integer arithmetic instead of floating point.
Example
use integer; $x = 10/3; # $x is now 3, not 3.33333333333333333
less
The less pragma is used to instruct perl to request less of a certain resource.
Example
use less 'memory'; use less 'CPU'; use less 'fat';
lib
The lib pragma is used to manipulate @INC at compile time.
Example
use lib LIST; no lib LIST;
locale
The locale pragma uses (or avoids) POSIX locales for built-in operations.
Example
@x = sort @y; # Unicode sorting order { use locale; @x = sort @y; # Locale-defined sorting order } @x = sort @y; # Unicode sorting order again
mro
The mro pragma defines method resolution order.
Example
use mro; # enables next::method and friends globally use mro 'dfs'; # enable DFS MRO for this class (Perl default) use mro 'c3'; # enable C3 MRO for this class
open
The open pragma sets default PerlIO layers for input and output.
Example
use open IN => ":crlf", OUT => ":bytes"; use open OUT => ':utf8'; use open IO => ":encoding(iso-8859-7)"; use open IO => ':locale'; use open ':encoding(utf8)'; use open ':locale'; use open ':encoding(iso-8859-7)'; use open ':std';
ops
The ops pragma restricts unsafe operations when compiling.
Example
perl -Mops=:default ... # only allow reasonably safe operations perl -M-ops=system ... # disable the 'system' opcode
overload
The overload pragma is used to overload perl operations.
Example
package SomeThing; use overload '+' => \&myadd, '-' => \&mysub; # etc ... package main; $a = SomeThing->new( 57 ); $b = 5 + $a; ... if (overload::Overloaded $b) {...} ... $strval = overload::StrVal $b;
overloading
The overloading pragma lexically controls overloading.
Example
{ no overloading; my $str = "$object"; # doesn't call stringification overload } # it's lexical, so this stringifies: warn "$object"; # it can be enabled per op no overloading qw(""); warn "$object"; # and also reenabled use overloading;
parent
The parent pragma establishes an ISA relationship with base classes at compile time.
Example
package Baz; use parent qw(Foo Bar);
re
The re pragma alters regular expression behavior.
Example
use re 'taint'; ($x) = ($^X =~ /^(.*)$/s); # $x is tainted here $pat = '(?{ $foo = 1 })'; use re 'eval'; /foo${pat}bar/; # won't fail (when not under -T # switch) { no re 'taint'; # the default ($x) = ($^X =~ /^(.*)$/s); # $x is not tainted here no re 'eval'; # the default /foo${pat}bar/; # disallowed (with or without -T # switch) } use re '/ix'; "FOO" =~ / foo /; # /ix implied no re '/x'; "FOO" =~ /foo/; # just /i implied use re 'debug'; # output debugging info during /^(.*)$/s; # compile and run time use re 'debugcolor'; # same as 'debug', but with colored # output ... use re qw(Debug All); # Same as "use re 'debug'", but you # can use "Debug" with things other # than 'All' use re qw(Debug More); # 'All' plus output more details no re qw(Debug ALL); # Turn on (almost) all re debugging # in this scope use re qw(is_regexp regexp_pattern); # import utility functions my ($pat,$mods)=regexp_pattern(qr/foo/i); if (is_regexp($obj)) { print "Got regexp: ", scalar regexp_pattern($obj); # just as perl would stringify } # it but no hassle with blessed # re's.
sigtrap
The sigtrap pragma enables simple signal handling.
Example
use sigtrap; use sigtrap qw(stack-trace old-interface-signals); # equivalent use sigtrap qw(BUS SEGV PIPE ABRT); use sigtrap qw(die INT QUIT); use sigtrap qw(die normal-signals); use sigtrap qw(die untrapped normal-signals); use sigtrap qw(die untrapped normal-signals stack-trace any error-signals); use sigtrap 'handler' => \&my_handler, 'normal-signals'; use sigtrap qw(handler my_handler normal-signals stack-trace error-signals);
sort
The sort pragma controls the behavior of sort().
Example
use sort 'stable'; # guarantee stability use sort '_quicksort'; # use a quicksort algorithm use sort '_mergesort'; # use a mergesort algorithm use sort 'defaults'; # revert to default behavior no sort 'stable'; # stability not important use sort '_qsort'; # alias for quicksort my $current; BEGIN { $current = sort::current(); # identify prevailing algorithm }
strict
The strict pragma restricts unsafe constructs.
Example
use strict; use strict "vars"; use strict "refs"; use strict "subs"; use strict; no strict "vars";
subs
The subs pragma pre-declares subroutine names.
Example
use subs qw(frob); frob 3..10;
threads
The threads pragma controls perl interpreter-based threads.
Example
use threads ('yield', 'stack_size' => 64*4096, 'exit' => 'threads_only', 'stringify'); sub start_thread { my @args = @_; print('Thread started: ', join(' ', @args), "\n"); } my $thr = threads->create('start_thread', 'argument'); $thr->join(); threads->create(sub { print("I am a thread\n"); })->join(); my $thr2 = async { foreach (@files) { ... } }; $thr2->join(); if (my $err = $thr2->error()) { warn("Thread error: $err\n"); } # Invoke thread in list context (implicit) so it can return a list my ($thr) = threads->create(sub { return (qw/a b c/); }); # or specify list context explicitly my $thr = threads->create({'context' => 'list'}, sub { return (qw/a b c/); }); my @results = $thr->join(); $thr->detach(); # Get a thread's object $thr = threads->self(); $thr = threads->object($tid); # Get a thread's ID $tid = threads->tid(); $tid = $thr->tid(); $tid = "$thr"; # Give other threads a chance to run threads->yield(); yield(); # Lists of non-detached threads my @threads = threads->list(); my $thread_count = threads->list(); my @running = threads->list(threads::running); my @joinable = threads->list(threads::joinable); # Test thread objects if ($thr1 == $thr2) { ... } # Manage thread stack size $stack_size = threads->get_stack_size(); $old_size = threads->set_stack_size(32*4096); # Create a thread with a specific context and stack size my $thr = threads->create({ 'context' => 'list', 'stack_size' => 32*4096, 'exit' => 'thread_only' }, \&foo); # Get thread's context my $wantarray = $thr->wantarray(); # Check thread's state if ($thr->is_running()) { sleep(1); } if ($thr->is_joinable()) { $thr->join(); } # Send a signal to a thread $thr->kill('SIGUSR1'); # Exit a thread threads->exit();
threads::shared
threads::shared perl extension for sharing data structures between threads.
Example
use threads; use threads::shared; my $var :shared; my %hsh :shared; my @ary :shared; my ($scalar, @array, %hash); share($scalar); share(@array); share(%hash); $var = $scalar_value; $var = $shared_ref_value; $var = shared_clone($non_shared_ref_value); $var = shared_clone({'foo' => [qw/foo bar baz/]}); $hsh{'foo'} = $scalar_value; $hsh{'bar'} = $shared_ref_value; $hsh{'baz'} = shared_clone($non_shared_ref_value); $hsh{'quz'} = shared_clone([1..3]); $ary[0] = $scalar_value; $ary[1] = $shared_ref_value; $ary[2] = shared_clone($non_shared_ref_value); $ary[3] = shared_clone([ {}, [] ]); { lock(%hash); ... } cond_wait($scalar); cond_timedwait($scalar, time() + 30); cond_broadcast(@array); cond_signal(%hash); my $lockvar :shared; # condition var != lock var cond_wait($var, $lockvar); cond_timedwait($var, time()+30, $lockvar);
utf8
The utf8 pragma enables/disables UTF-8 (or UTF-EBCDIC) in source code.
Example
use utf8; no utf8; # Convert the internal representation of a Perl scalar to/from UTF-8. $num_octets = utf8::upgrade($string); $success = utf8::downgrade($string[, FAIL_OK]); # Change each character of a Perl scalar to/from a series of # characters that represent the UTF-8 bytes of each original character. utf8::encode($string); # "\x{100}" becomes "\xc4\x80" utf8::decode($string); # "\xc4\x80" becomes "\x{100}" $flag = utf8::is_utf8(STRING); # since Perl 5.8.1 $flag = utf8::valid(STRING);
vars
The vars pragma pre-declares global variable names.
Example
use vars qw($frob @mung %seen);
vmsish
The vmsish pragma controls VMS-specific language features.
Example
use vmsish; use vmsish 'status'; # or '$?' use vmsish 'exit'; use vmsish 'time'; use vmsish 'hushed'; no vmsish 'hushed'; vmsish::hushed($hush); use vmsish; no vmsish 'time';
warnings
The warnings pragma controls optional warnings.
Example
use warnings; no warnings; use warnings "all"; no warnings "all"; use warnings::register; if (warnings::enabled()) { warnings::warn("some warning"); } if (warnings::enabled("void")) { warnings::warn("void", "some warning"); } if (warnings::enabled($object)) { warnings::warn($object, "some warning"); } warnings::warnif("some warning"); warnings::warnif("void"", "some warning"); warnings::warnif($object, "some warning");
warnings:register
The warnings::register is the warnings import function.
Example
use warnings::register;