Polymorphism
Updated: 06/30/2019 by Computer Hope
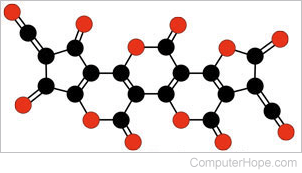
In computer science, polymorphism refers to the ability of a programming language to interpret objects in different ways based on their class or data type. In essence, it is the ability of a single method to be applied to derived classes and achieve a proper output.
Tip
You can remember this word by breaking it down. The word "poly" means "many" and "morph" means "form."
Three branches of polymorphism
- Ad hoc polymorphism is when a function is implemented differently depending on a limited number of specified types and combinations of input parameters. An example of ad hoc polymorphism is function overloading.
- Parametric polymorphism is when code is written without any specification of type, and so can be used with any number of different types specified later. In object-oriented programming, this is often called generic programming.
- Inclusion polymorphism, also known as subtyping, is when a single name refers to many instances of different classes as long as they share the same superclass.
Polymorphism example in Java
Example code
class OverloadedObject { void overloadedMethod (int a) { System.out.println("This method is called when the parameter is an integer. a = " + a); } void overloadedMethod (int a, int b) { System.out.println("This method is called when there are two parameters, and they are both integers. a = " + a + ", b = " + b); } void overloadedMethod (double a) { System.out.println("This method is called when there is one parameter, and it is a double-precision number. a = " + a); } } class DemonstrateOverloading { public static void main (String args []) { OverloadedObject myObj = new OverloadedObject(); myObj.overloadedMethod(1); myObj.overloadedMethod(2, 3); myObj.overloadedMethod(4); } }
Example code output
This method is called when the parameter is an integer. a = 1 This method is called when there are two parameters, and they are both integers. a = 2, b = 3 This method is called when the parameter is a double-precision number. a = 4
Notice that it produces different output based on different input parameters, even though the class and method name invoked are the same each time.
Object-oriented, Output, Parameter, Polymorphic virus, Programming language, Programming terms